Introduction to Scriptable Objects
Tutorial
·
Beginner
·
+10XP
·
65 mins
·
(402)
Unity Technologies
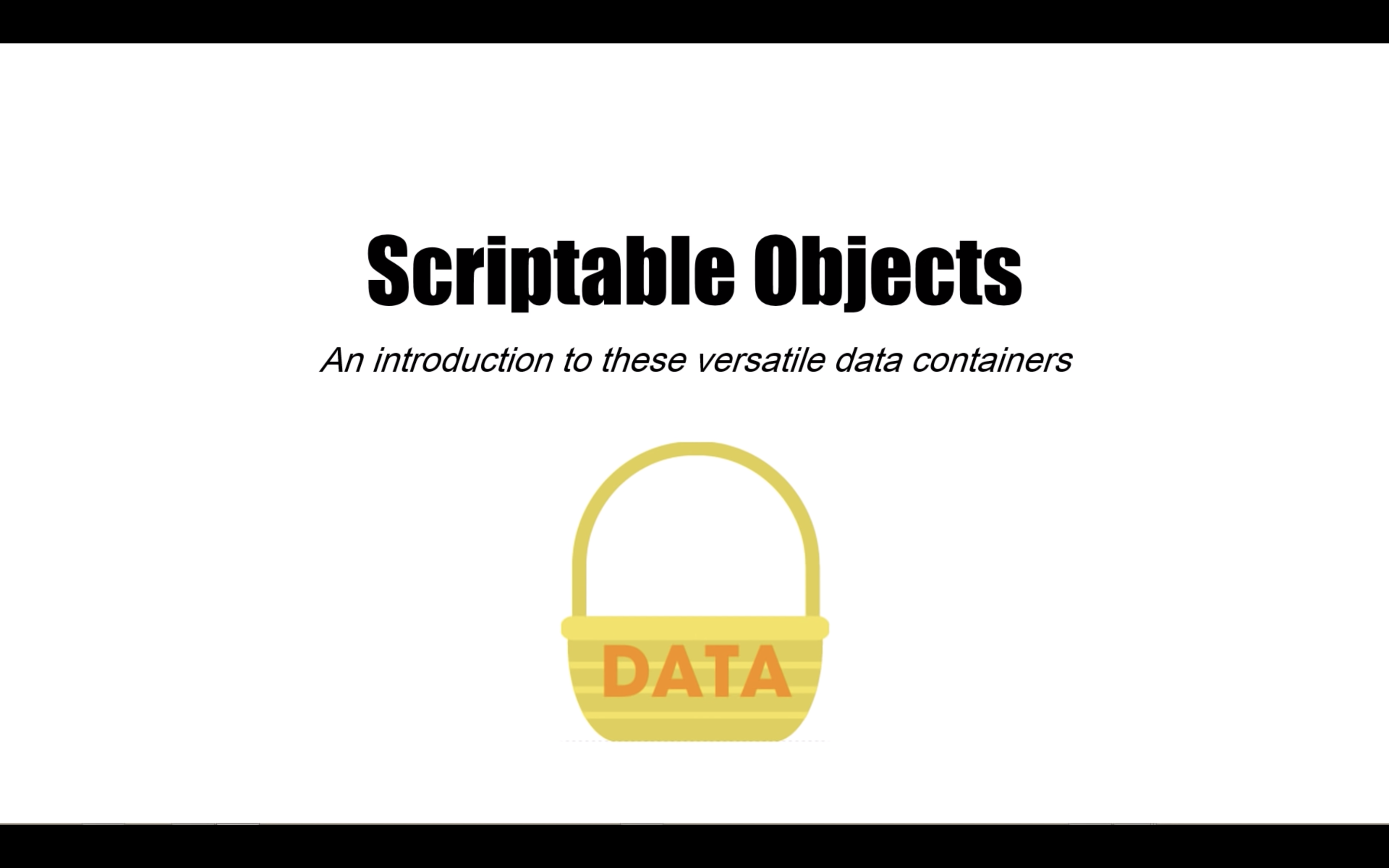
Languages available:
Tutorial complete!
+10 XP
You gained 10 XP points towards
How would you rate this tutorial?
Tutorial
·
Beginner
·
+10XP
·
65 mins
·
(402)
Unity Technologies
Languages available:
+10 XP
You gained 10 XP points towards